Recently, I was trying to generate line art from Ken Sugimori Pokémon artwork, like this Turtwig here:
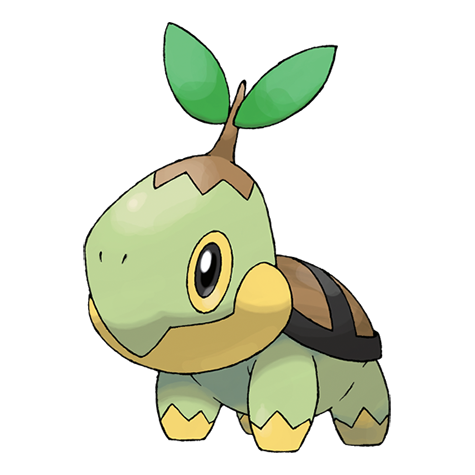
I came across this site which did a pretty good job out of the box, with some more options for customisation:
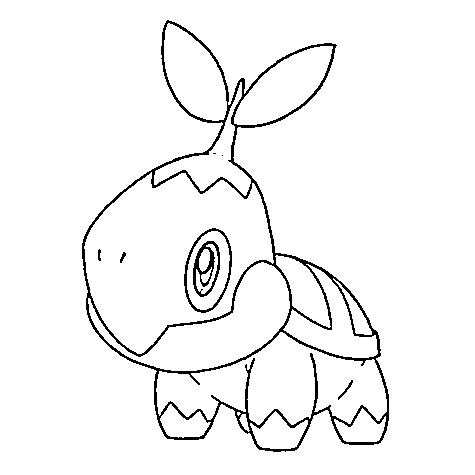
Could we do something similar be done with ImageMagick?
Preprocessing
To make things easier for our edge detection algorithms, let's preprocess our image by converting it to grayscale and remove the alpha channel.
The following command does this and saves the output to the non-portable ImageMagick-specific Magick Persistent Cache intermediate format (search for “MPC” at Supported image formats):
convert turtwig.png -colorspace gray -alpha remove turtwig-preprocessed.mpc
This will create two files, turtwig-preprocessed.mpc
and turtwig-preprocessed.cache
.
Without removing the alpha channel, it seems that most edge detection techniques (which mainly look for sudden changes in colour) only return Turtwig's outline, presumably because the alpha values suddenly go from 0% in the background to 100% on Turtwig:
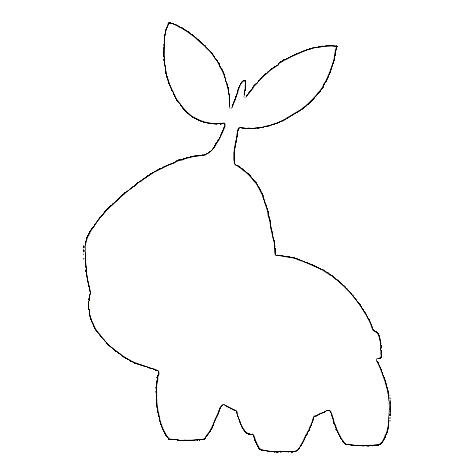
Convolution
Before deep learning and convolutional networks became mainstream, a similar technique with image kernels was already in use for image processing. Effects such as blurring, sharpening and embossing—which some may have first encountered in image editing software—are applied by performing a convolution with an appropriate kernel.
Edge detection is just another effect, and there are specific kernels which can be used to do this, for example the Sobel operator and Roberts cross, as well as algorithms involving kernels such as the Difference of Gaussians.
ImageMagick supports convolution and the ImageMagick usage examples contain a section on Edge Detection Convolutions, which the following snippets are based on.
Difference of Gaussians
convert turtwig-preprocessed.mpc \
-morphology Convolve DoG:1,0,1 \
-negate \
-tint 0 \
turtwig_laplacian_isotropic.png
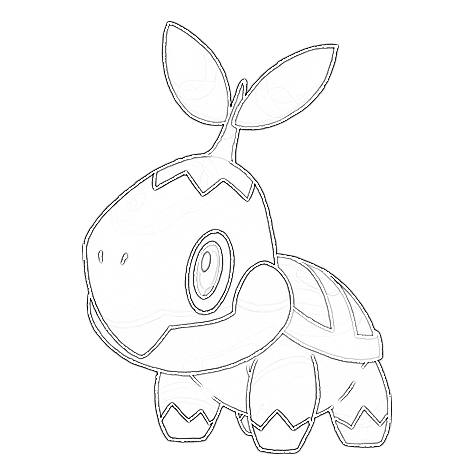
Discrete Laplacian kernels
convert turtwig-preprocessed.mpc \
-define convolve:scale='!' \
-morphology Convolve Laplacian:0 \
-negate \
-tint 0 \
turtwig_laplacian_0.png
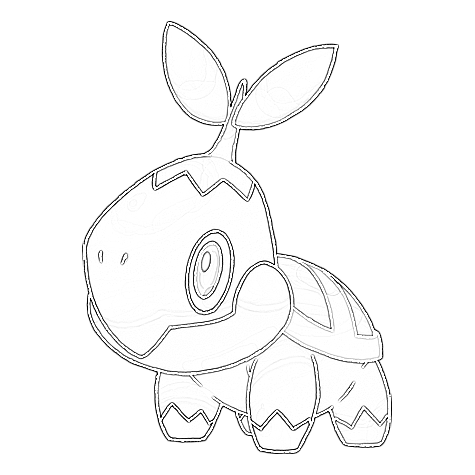
Sobel operator
convert turtwig-preprocessed.mpc \
-define convolve:scale='50%!' \
-bias 50% \
\( -clone 0 -morphology Convolve Sobel:0 \) \
\( -clone 0 -morphology Convolve Sobel:90 \) \
-delete 0 \
-solarize 50% \
-level 50,0% \
-compose Lighten \
-composite \
-negate \
turtwig_sobel_maximum_3.png
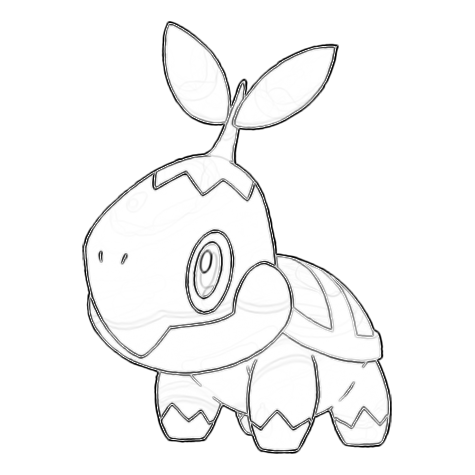
Roberts cross
convert turtwig-preprocessed.mpc \
-define morphology:compose=Lighten \
-morphology Convolve 'Roberts:@' \
-negate \
turtwig_roberts_maximum.png
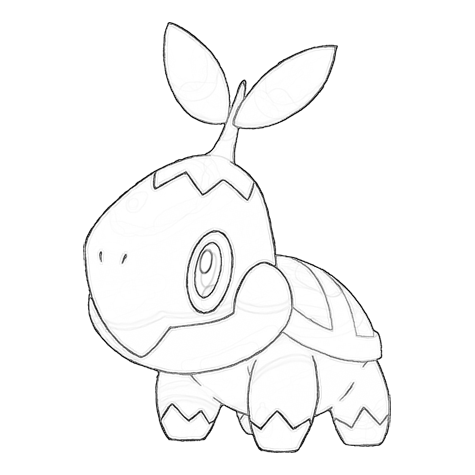
Combining the Roberts cross with a Gaussian blur and the -auto-threshold
option seems to provide the best
results:
convert turtwig-preprocessed.mpc \
-define morphology:compose=Lighten \
-morphology Convolve 'Roberts:@' \
-negate \
-gaussian-blur 1x1 \
-auto-threshold OTSU \
turtwig_roberts_maximum_threshold.png
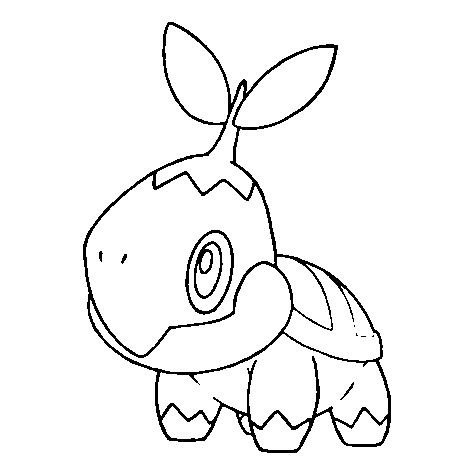
Computer vision transformations
Turns out that as an alternative to fiddling with image kernels, ImageMagick also provides some built-in line detection algorithms as well. These are shown under Computer Vision Transformations.
Edge detection
convert turtwig-preprocessed.mpc -negate -edge 1 -negate turtwig-edge.png
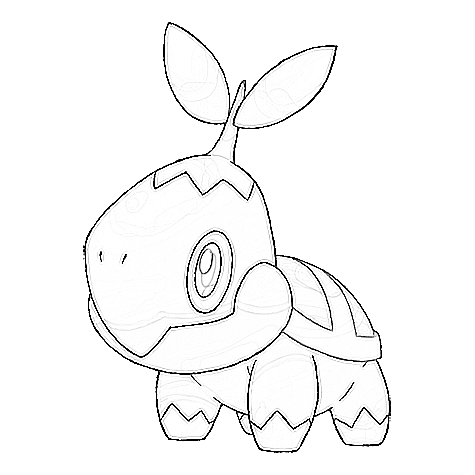
The double negation avoids “twinning” in the detected edges.
Canny edge detector
The Canny edge detector is an advanced, multi-stage algorithm for edge detection.
convert turtwig-preprocessed.mpc -canny 0x1+10%+30% -negate turtwig-canny.png
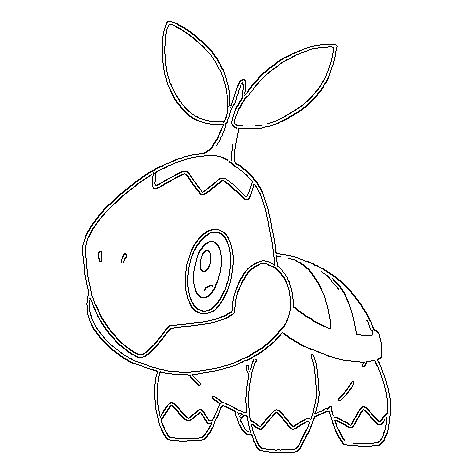
Bonus: Colour masking
It just happens that Sugimori Pokémon images already happen to have nice black lines already. What if we just removed every pixel which wasn't black (source)?
convert turtwig.png \
-matte \( +clone -fuzz 15% -transparent black \) \
-compose DstOut \
-composite \
turtwig_lines.png
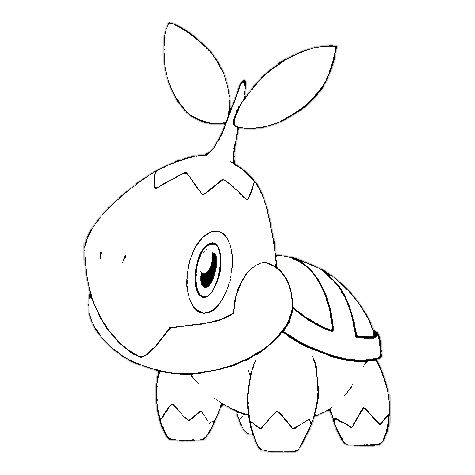
I guess it kind of works?